Риддик / Riddick
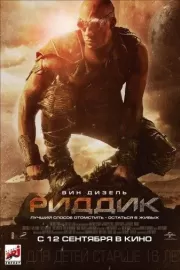
- Ограничение: 16++
- Год выхода: 2013
- Страна: США, Великобритания
- Жанр: Фантастика, Блокбастер, Боевик, Триллер, Зарубежный
- Режиссер: Дэвид Туи
- Актеры: Вин Дизель, Хорди Молья, Мэтью Нэйбл, Кэти Сакхофф, Дэйв Батиста, Боким Вудбайн, Рауль Макс Трухильо, Конрад Пла, Дэнни Бланко, Карл Урбан
Сюжет:

Поиск торрент раздач, пожалуйста подождите!
Первоначальное название сценария было — «Хроники Риддика: Преследование мертвеца» (The Chronicles of Riddick: Dead Man Stalking). Кери Хилсон проходила прослушивание на роль Дали. Роль в итоге отошла Кэти Сакхофф, но Дэвид Туи был настолько впечатлен харизмой Хилсон, что написал небольшую роль специально для нее. Производство фильма оказалось под угрозой срыва, когда была задержка в финансировании. В итоге Вин Дизель взял на себя финансирование фильма до тех пор, пока не разрешилась проблема с банковской ссудой. Вин Дизель согласился сыграть эпизодическую роль в фильме «Тройной форсаж: Токийский Дрифт» (2006) в обмен на права на франшизу «Риддика» от Universal Pictures. Это позволило ему заниматься производством «Риддика» самостоятельно. Персонаж Мэтью Нэйбла — Босс Джонс — это отец Уильяма Дж. Джонса, которого в фильме «Черная дыра» (1999) сыграл Коул Хаузер. В реальной жизни Нэйбл всего на три года старше Хаузера.
Комментарии (0)